What is work-stealing in Java?
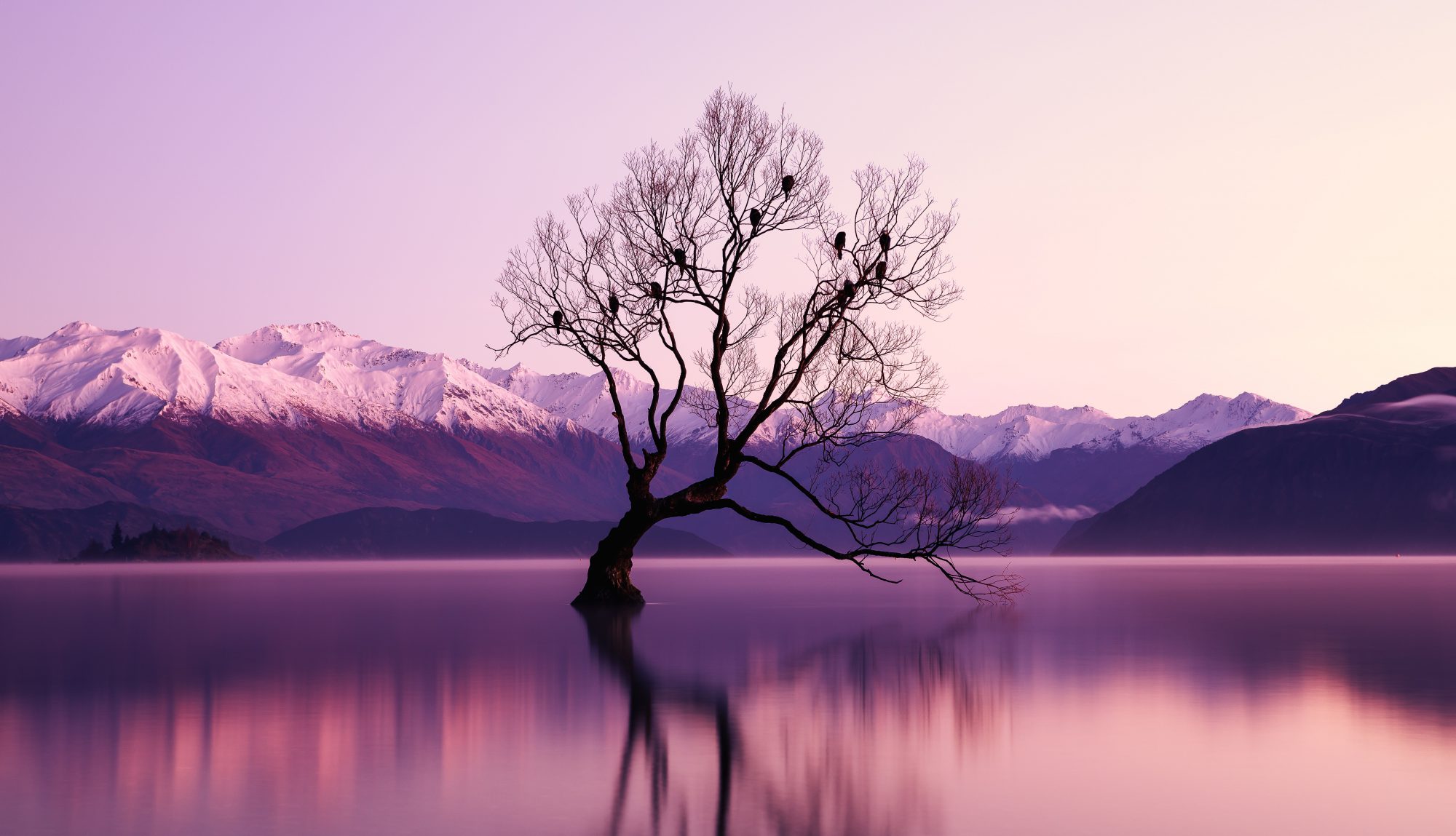
What is work-stealing in Java?
Work stealing is a scheduling strategy where worker threads that have finished their own tasks can steal pending tasks from other threads. In parallel execution, tasks are divided among multiple processors/cores.
How do you make work-stealing thread pool?
We can create a work-stealing thread pool using either the ForkJoinPool class or the Executors class: ForkJoinPool commonPool = ForkJoinPool. commonPool(); ExecutorService workStealingPool = Executors.
What is a work-stealing queue?
A work-stealing queue enables one thread (queue owner) to push/pop items into/from one end of the queue, and multiple threads (thieves) to steal items from the other end.
How does ThreadPoolExecutor work in Java?
To use thread pools, we first create a object of ExecutorService and pass a set of tasks to it. ThreadPoolExecutor class allows to set the core and maximum pool size. The runnables that are run by a particular thread are executed sequentially.
What does fork () Do Java?
Fork/Join in Java is used to make use of the cores (brain of CPU that process the instructions) in an efficient manner. The fork/join splits a bigger task into smaller sub-tasks. These sub-tasks are then distributed among the cores. The results of these subtasks are then joined to generate the final result.
How does fork join pool work?
The design of ForkJoinPool is actually very simple, but at the same time it’s very efficient. It’s based on the “Divide-And-Conquer” algorithm; each task is split into subtasks until they cannot be split anymore, they get executed in parallel and once they’re all completed, the results get combined.
Which feature of Java 8 enables us to create a work-stealing thread pool using all available processes at its target?
Which feature of java 8 enables us to create a work stealing thread pool using all available processors at its target? Explanation: Executors newWorkStealingPool() method to create a work-stealing thread pool using all available processors as its target parallelism level.
Is ThreadPoolExecutor thread safe?
For ThreadPoolExecutor the answer is simply yes. ExecutorService does not mandate or otherwise guarantee that all implementations are thread-safe, and it cannot as it is an interface. These types of contracts are outside of the scope of a Java interface.
How do you fork join a pool in Java?
Java ForkJoinPool Class Example: invoke()
- import java.util.Random;
- import java.util.concurrent.ForkJoinPool;
- import java.util.concurrent.RecursiveTask;
- public class JavaForkJoinPoolinvokeExample1 extends RecursiveTask {
- private static final int var = 5;
- private final int[] value;
- private final int st;
What is executor framework How is it different from fork join framework?
In short, the main difference between the Executor framework and ForkJoinPool is that the former provides a general-purpose thread pool, while the latter provides a special implementation that uses a work-stealing pattern for efficient processing of ForkJoinTask.
What is ForkJoinPool commonPool ()?
commonPool. public static ForkJoinPool commonPool() Returns the common pool instance. This pool is statically constructed; its run state is unaffected by attempts to shutdown() or shutdownNow() . However this pool and any ongoing processing are automatically terminated upon program System.
What is difference between executor framework and ForkJoinPool?
How many threads does fork join use?
Its implementation restricts the maximum number of running threads to 32767 and attempting to create pools with greater than this size will result to IllegalArgumentException . The level of parallelism can also be controlled globally by setting java.
What are the features of Java 8 with example?
Top Java 8 Features With Examples
- Functional Interfaces And Lambda Expressions.
- forEach() Method In Iterable Interface.
- Optional Class.
- Default And Static Methods In Interfaces.
- Java Stream API For Bulk Data Operations On Collections.
- Java Date Time API.
- Collection API Improvements.
- Java IO Improvements.
Is set thread-safe Java?
You should not access the original set directly. This means that no two methods of the set can be executed concurrently (one will block until the other finishes) – this is thread-safe, but you will not have concurrency if multiple threads are using the set.
Is Python ThreadPoolExecutor thread-safe?
ThreadPoolExecutor Thread-Safety Although the ThreadPoolExecutor uses threads internally, you do not need to work with threads directly in order to execute tasks and get results. Nevertheless, when accessing resources or critical sections, thread-safety may be a concern.
What is work stealing in Linux?
Work stealing is a scheduling strategy where worker threads that have finished their own tasks can steal pending tasks from other threads. In parallel execution, tasks are divided among multiple processors/cores.
What is work stealing in parallel execution?
Work stealing is a scheduling strategy where worker threads that have finished their own tasks can steal pending tasks from other threads. In parallel execution, tasks are divided among multiple processors/cores. When a core has no work, it should be assigned a task from another processor’s overloaded queue rather than being idle.
How does a worker thread steal work from another thread?
At this point, the worker thread randomly selects a peer thread-pool thread it can “steal” work from. It then uses the first-in, first-out approach (FIFO) to take sub-tasks from the tail end of the victim’s deque. 3. Fork/Join Framework Implementation
Does task granularity affect the level of work stealing?
The level of work stealing is always high and not influenced much by the change in task granularity. Technically speaking, our prime numbers example is one that supports asynchronous processing of event-style tasks. This is because our implementation does not enforce the joining of results.